Structure
2.1 Introduction
2.2 Selection control statements in C++
Self Assessment Questions
2.3 Iteration statements in C++
Self Assessment Questions
2.4 Break, continue and exit statements in C++
Self Assessment Questions
Summary
Terminal Questions
2.1 Introduction
This unit has the following objectives
· To learn to use selection control statements in C++
· To learn to use loops or iteration in C++
· To implement programs using relational, logical, increment and decrement operators in C++
2.2 Selection control statements in C++
There are basically two types of control statements in c++ which allows the programmer to modify the regular sequential execution of statements.They are selection and iteration statements. The selection statements allow to choose a set of statements for execution depending on a condition. If statement and switch statement are two statements which allow selection in c++. There is also an operator known as conditional operator which enables selection.
If statement
Syntax : if (expression or condition)
{ statement 1;
statement 2;
}
else
{ statement 3;
statement 4;
}
The expression or condition is any expression built using relational operators which either yields true or false condition. If no relational operators are used for comparison, then the expression will be evaluated and zero is taken as false and non zero value is taken as true. If the condition is true, statement1 and statement2 is executed otherwise statement 3 and statement 4 is executed. Else part in the if statement is optional. If there is no else part, then the next statement after the if statement is exceuted, if the condition is false. If there is only one statement to be executed in the if part or in the else part, braces can be omitted.
Following example program implements the if statement.
// evenodd.cpp
# include <iostream.h>
# include <conio.h>
void main()
{
int num;
cout<<”Please enter a number”<<endl;
cin>>num;
if ((num%2) == 0)
cout<<num <<” is a even number”;
else
cout<<num <<” is a odd number”;
getch();
}
The above program accepts a number from the user and divides it by 2 and if the remainder (remainder is obtained by modulus operator) is zero, it displays the number is even, otherwise as odd. We make use of the relational operator == to compare whether remainder is equal to zero or not.
Nested If statement
If statement can be nested in another if statement to check multiple conditions.
If (condition1)
{ if (condition 2)
{ statement1;
Statement2;
}
else if (condition3)
{statement3;
}
}
else statement4;
The flowchart of the above example is shown below
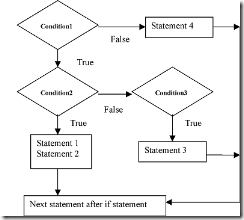
Fig. 2.1: Nested If Statement
Multiple conditions can be checked using logical && operator(AND) and || operator (OR).
If ((condition1) && (condition2))
statement1;
else
statement2;
In the above example statement1 will be executed if both the condition1 and condition2 are true and in all other cases statement2 will be executed.
If ((condition1 || (condition2))
statement1;
else
statement2;
In the above example statement1 will be executed if either condition1 or condition2 are true and even if both are true. Statement2 will be executed if both the conditions are false. The following program demonstrates the use of && operator and nested if statement.
//Large.cpp
# include <iostream.h>
void main()
{ int a,b,c;
cout<<”Please enter three numbers”;
cin>>a>>b>>c;
if ((a>b) && (b>c))
cout<<a<< “ is the largest number”;
else if ((b>a) && (b>c))
cout<<b<< “ is the largest number”;
else if ((c>a) && (c>b))
cout<<c<< “ is the largest number”;
}
The above program accepts three numbers from the user and displays which is the largest number among the three.( assumption is that all the numbers are unique, the program has to be modified if you would like to allow same number twice)
Switch statement
Nested ifs can be confusing if the if statement is deeply nested. One alternative to nested if is the switch statement which can be used to increase clarity in case of checking the different values of the same variable and execute statements accordingly.
Syntax :
Switch (variablename)
{ case value1: statement1;
break;
case value2: statement2;
break;
case value3: statement3;
break;
default: statement4;
}
If the variable in the switch statement is equal to value1 then statement1 is executed, if it is equal to value2 then statement2 is executed, if it is value3 then statement3 is executed. If the variable value is not in any of the cases listed then the default case statement or statement4 is executed. The default case specification is optional, however keeping it is a good practice. It can also be used for displaying any error message. Each case can have any number of statements. However every case should have a break statement as the last statement. Break statement takes the control out of the switch statement. The absence of the break statement can cause execution of statements in the next case. No break is necessary for the last case. In the above example, default case does not contain a break statement.
The flowchart for the switch statement is shown in Fig 2.2
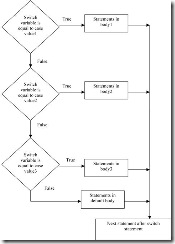
Fig. 2.2: Switch Statement
The following program implements the switch statement
position.cpp
# include<iostream.h>
void main()
{ char pos;
int x=15, y=15;
cout << “ you are currently located at” <<x<<” “<<y<<endl;
cout>>”please choose the letter to move l for left, r for right, u for up and d for down” <<endl;
cin>>pos;
switch (pos)
{ case ‘l’: x--;
break;
case ‘r’: x++;
break;
case ‘u’: y++;
break;
case ‘d’: y--;
break;
default: cout<<”You selected a wrong option”;
}
cout<<“ you are now located at” <<x<<” “<<y;
}
The above program asks the user to enter l,r,u,d for allowing him to move left,right,up and down respectively. The position is initialised to 15 and 15 which are x and y coordinates of his position. Depending upon the what user has selected the the x and y co-ordinates are incremented or decremented by one(x++ is same as x=x+1). If the user types a letter other than l,r,u,d, he gets an error message. Since the switch variable is a character, l,u,r,d and enclosed within single quote.
++ and -- operator can be used as postfix or as prefix operator which has no effect if used as an independent statement. However if it used as part of an expression, the prefix operator will be operated and then the expression will be evaluated whereas the postfix operated will be evaluated later.
For example in the statement x= a+ (b++), a will be added to b and then stored in x and then the value of b will be incremented. If the same expression is written as x=a+(++b), the the b will be incremented and then added to a and stored in x.
Conditional Operator
Conditional operator (?:) is a handy operator which acts like a shortcut for if else statement. If you had to compare two variables a and b and then depending on which is larger, you wanted to store that variable in another variable called large. You would do this using if else statement in the following way:
if (a>b)
large=a;
else
large=b;
The above can be done using conditional operator in the following way:
large= (a>b) ? a : b ;
Self Assessment Questions
1. In if else statement, at any point of time statements in both the if and else part may be executed. True/False
2. Conditional operator is an alternative to __________ statement
3. ++ operator increments the value of the operand by _________
4. The ++ operator used as postfix and prefix has the same effect. True or False
5. Each case in switch statement should end with _________ statement.
2.3 Iteration statements in C++
Iteration or loops are important statements in c++ which helps to accomplish repeatitive execution of programming statements. There are three loop statements in C++ : while loop, do while loop and for loop
While loop
Syntax: while (condition expression){
Statement1;
Statement 2;
}
In the above example, condition expression is evaluated and if the condition is true, then the statement1 and statement2 are executed. After execution, the condition is checked again. If true, the statements inside the while loop are executed again. This continues until the loop condition becomes false. Hence the variable used in the loop condition should be modified inside the loop so that the loop termination condition is reached. Otherwise the loop will be executed infinitely and the program will hang when executed. Also the loop variable should be iniatialised to avoid the variable taking junk value and create bugs in the program.
The flowchart for the While statement is shown in Fig 2.3.
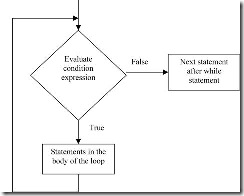
Fig. 2.3: While Statement
The following program implements the while loop
// average1.cpp
# include <iostream.h>
void main()
{
int n=0,a;
int sum=0;
cout<< “enter five numbers”;
while (n<5)
{
cin>>a;
sum=sum+a;
n++;
}
cout<<”Average of the numbers is”<<(sum/n);
}
The above program accepts five numbers from the user and finds the average of the five numbers. In the above while loop, the variable n is initialized to zero and this variable keeps track of the count of numbers input from the user. It is incremented every time a number is input from the user. The number accepted from the user is added to the value stored in the sum variable. When n becomes 5 the while loop condition becomes false and the average of the numbers is printed. Please note that there is no semicolon after while and condition expression.
Do..while loop
The do while loop is same as while loop except that the condition is checked after the execution of statements in the do..while loop. Hence in do..while loop, statements inside the loop are executed atleast once. However, in while loop, since the condition is checked before, the statements inside the loop will not be executed if the loop condition is false.
Syntax:
do
{
Statement1;
Statement2
} while (condition expression);
In the above example the statement1 and statement2 are executed and the condition is checked. If the condition is true then the statements are executed again and if the condition is false then the control is transferred to the next statement after the do..while statement.
The flowchart for the do..while statement is shown in Fig 2.4
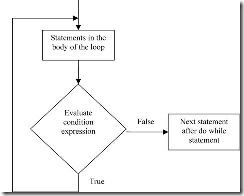
Fig. 2.4: Do..while Statement
Please note that there is no semicolon after do, but there is a semicolon after the condition expression in while part. The average1.cpp program is rewritten using do..while loop as follows
// average2.cpp
# include <iostream.h>
void main()
{
int n=0,a;
int sum=0;
cout<< “enter five numbers”;
do
{
cin>>a;
sum=sum+a;
n++;
}while (n<5);
cout<<”Average of the numbers is”<<(sum/n);
}
The decision on whether to use while or do..while statement depends on whether the statements inside the loop have to be executed atleast once or not. If it has to be executed atleast once, then the do..while statement should be used.
For loop
The for loop is one of the popular control statement as it is compact and clear in specification. The loop initialization, loop termination condition statement and statement for the next iteration are all included in one statement.Syntax:
for(initialization statement;loop termination condition;statement to increment/decrement the loop variable)
{
Statement1;
Statement2;
}
In the above example, initiation statement is executed first and then the termination is checked. If the condition is true, statement1 and statement2 will be executed. Then the third statement in the for loop is executed which modifies the loop control variable.
The flowchart for the for statement is shown in Fig 2.5
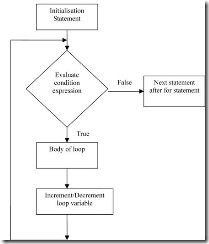
Fig. 2.5: For Statement
Please note that there is no semicolon after the for statement.
The average2.cpp rewritten using for loop is as follows:
// average3.cpp
# include <iostream.h>
void main()
{
int a;
int sum=0;
cout<< “enter five numbers”;
for(int n=0;n<5;n++)
{
cin>>a;
sum=sum+a;
}
cout<<”Average of the numbers is”<<(sum/n);
}
In the above example n variable is loop variable which is initialized to zero. Please note that the variable is also declared inside the for loop. So this variable can be accessible only inside the for loop. The scope of the variables will be declared in detail in unit 4. The statements inside the loop are executed until the loop termination condition n<5 becomes false. After every iteration, the loop variable n is incremented.
The following program demonstrates the use of for loop using decrement operator to find the factorial of a number.
// factorial.cpp
# include <iostream.h>
void main()
{
int number,fact=1;
cout<< “enter a number”;
cin>>number;
for(int i=number;i>1;i--)
fact=fact*i;
cout<<”Factorial of <<number<<“is”<<fact;
}
All the above loops can be nested. The inner loop is executed completely and then the outer loop is executed. For every iteration of outer loop, all the iterations of the inner loop is executed.
The for statement can also have multiple initialization and decrement and increment statements as shown in the following expression
for(i=0,j=0;i<=n;j++,i++)
{
statements;
}
Please note that in case of multiple statements, each statement should be separated by a comma.
Selection of which type of loop to be used depends on the programmer and his style. However you should use for loop if you know in advance how many times the loop has to be executed. If the loop has to be executed atleast once irrespective of whether the condition is true or false, then do while loop should be used.
Self Assessment Questions
1. Which of the following loop will be executed atleast once even if the condition is false.
a. For loop b. while loop c. do..while loop d. None of the above
2. ___________ is the loop variable in the loop for(int k=n;k!=0;k=k-2)
3. The loop for(int i=0;i<n;i++) will be executed till the statement _________ becomes false.
4. If there is more than one statements inside a loop, then the statement should be enclosed within ______________
2.4 Break, continue and exit statements in C++
All the loops or iteration statements discussed in previous section are executed until the loop condition becomes false. However if required, the control sequence can be changed by using break, continue or exit statements.
Break statement transfers the control to the next statement after the loop and all the remaining statements and the iterations in the loop are skipped. It is used if you would like to exit the loop when a certain condition is met. In the switch statement break statement takes the control to the next statement after the switch statement.
The following program demonstrates the use of break statement inside for loop.
// prime.cpp
# include <iostream.h>
void main()
{ int number;
int prime=0;
cout<<”Enter a number”;
cin>>number;
// check whether the number is divisible by any number from 2 to number/2
for (int i=2;i<=number/2;i++)
{
if ((number%i)==0)
{ prime=1;
break;
}
}
if (prime==0)
cout<<”The number is prime number”;
else
cout<<” The number is not a prime number”;
}
In the above program, the user is asked to enter a number and the program checks whether the number is prime or not and displays the message accordingly. To check whether the number is prime or not, the number is divided by 2,3… and so on till number/2. If the number is divisible by any number, the variable prime is assigned a value one and the loop is terminated as there is no need to check whether the number is divisible by rest of the numbers. The value 1 in the prime indicates that the number was divisible by one of the numbers between 2 to number/2.
Continue statement is used to take the control to the next iteration of the loop. It is used if the control has to be transferred to the next iteration of the loop based on a condition skipping all the other statements in the loop. The following program shows the use of continue statement.
move.cpp
# include<iostream.h>
# include<conio.h>
void main()
{ int x,y;
char choice=’y’;
do
{
clrscr();
cout<<”please enter the dividend”;
cin>>x;
cout<<”Please enter the divisor”;
cin>>y;
if (y==0)
{
cout<<”error!! Division by zero, try again”;
continue;
}
cout<<”The quotient is “<<(x/y);
cout<<”the remainder is”<<(x%y);
cout<<”do u wish to continue”;
cin>>choice;
}while (choice==’y’);
}
In the above example clrscr() function which is a library function is used to clear the screen before accepting the input from the user. To use this function, conio.h header file must be included.
Exit function in a program takes the control out of the program. Exit is an inbuilt library function. To use this function process.h header file has to be included in the program. The syntax of this function is exit(integer).While terminating the program, the integer is returned to the operating system. Usually the function integer 0 is used to imply no error or successful termination and non zero value implies error while exiting the program .
Go to statement is a statement which should be avoided. It take the control to a particular statement. The statement should have a label.
Syntax: goto statement labelname
Statements can be given labels in the following way
labelname: statement
The use of goto statement should be avoided as it creates problems in program debugging and the program may have confusing jumps from one statement to another.
Self Assessment Questions
1. _________ statement takes the control out of the loop.
2. __________ header file should be included to use exit function
3. __________ statement takes the control to the beginning of the loop.
4. ________ statement takes the control to a particular statement
Summary
Selection and iteration statements allow to execute statements in the program based on a condition bypassing the normal sequential execution. Selection is enabled through if statement, switch statement and conditional operator. Switch statement is suitable when you have multiple conditions to be checked and nested if can be avoided. Iteration is enabled in C++ through while, do..while and for loops. Loops execute the statements repeatitively until a particular condition becomes false. Break, continue and exit statements are used to transfer control out of the loop, to the next iteration of the loop and out of the program respectively.
Terminal Questions
1. Write a program which accepts a number from the user and generates prime numbers till that number
2. Write a program that accepts a number ‘n’ from the user and generates Fibonacci series till n (Fibonacci series starts with 0 and 1 and then the subsequent numbers are generated by adding the two previous numbers in the series.
3. The loop for(j=0;j<100;j=j+2) will be executed how many times
a) 100 b) 50 c) 51 d) 49
4. The loop
x=10;
do {
cout<<x;
x++;
}
while (x<10) will be executed how many times
a) 1 b) 10 c)0 d)9
5. Write the output of the following C++ code
# include<iostream.h>
void main()
{ int x=1;
switch (x)
{
case 0 : cout<<”zero”;
break;
case 1: cout << “one”;
case 2: cout<<”two”;
}
}
Answers (SAQs and TQs)
Saqs in 2.2
1. False
2. if statement
3. one value
4. False
5. break statement.
Saqs in 2.3
1. do..while loop
2. k
3. i<n
4. statements should be enclosed in flower brackets { }
Saqs in 2.4
1. break
2. process.h
3. continue
4. go to
TQs
1. // primegen.cpp
# include <iostream.h>
void main()
{ int number;
int prime;
cout<<”Enter a number”;
cin>>number;
for(int i=2;i<=number;i++)
{prime=0;
for (int j=2;j<=i/2;j++)
{
if ((i%j)==0)
{ prime=1;
break;
}
}
if (prime==0)
cout<<i <<endl;
}
}
2. // fibonacci.cpp
# include <iostream.h>
void main()
{ int number;
int i=0,j=1,k;
cout<<”Enter the upper limit of the series”;
cin>>number;
cout<<” the Fibonacci series till ”<<number <<”is”<<endl;
if (number<0)
cout<<”invalid number”;
else if (number==0)
cout<<i;
exit(0);
else
{cout<<i<<endl<<j<<endl;
k=i+j;
do
{
cout<< k<<endl;
k=i+j;
i=j;
j=k;
}while (k<=number);
}
}
3. b
4. a
I have 2 condition & 3 code block
ReplyDeletescenario 1:
if condition1 {block1}
if condition2 {block2}
if condition1 OR condition2 {block3}
optimising:
if condition1 OR condition2 {
block3
if condition1 {block1}
else {block2}
}
scenario 2:
if condition1 {block1}
if condition2 {block2}
if condition1 AND condition2 {block3}
w/o store Bool or Any
in scenario 1-> 2*(2 condition) = 4 time check in general
by optimising 2*(1 condition) + 1 = 3 time check
any other trick that do this in 2 time check (each one 1 time only)
in scenario 2-> 2*(2 condition) = 4 time check in general
any other trick that do this in 3/2 time check
optimising scenario 2:
Deleteif condition1{
block1
if condition2 {block2 block3}
}
else if condition2{block2}
reduce condition check
but code block increase
so this is bad
optimising scenario 1:
Deletein function : - - -
if condition1 {block1}
else if condition2 {block2}
else {return}
block3
reduce condition check more only 2
but need to create function
so this is -_-!
This comment has been removed by the author.
ReplyDelete