Unit 5 Objects and Classes
Structure
5.1 Introduction
5.2 Creating classes and objects
Self Assessment Questions
5.3 Constructors and Destructors
Self Assessment Questions
5.4 Static variables and Functions in class
Self Assessment Questions
Summary
Terminal Questions
5.1 Introduction
This unit has the following objectives
· To understand what are classes and Objects and learn to implement them
· To learn to use constructors and destructors in a class
· To understand static variables and functions with respect to a class
5.2 Creating classes and objects
In the unit 1, we had a brief discussion of objects and classes. In this section, we will see how we can implement them. Classes provide users a method to create user defined datatypes. We have seen two types of user defined datatypes structures and enumeration in the last unit. Both are primarily used for modeling data only. In several real life situation, you would require to model functionality of the datatype along with the data. Classes enable you to bind data and functions that operate on data together. However, in C++ you can use structures same as classes by binding functions with data. But usually the structures are only used when you want to group data and classes when you want to group both data and functions.
Classes can model either physical or real things such as employee, product, customer etc or it can model a new datatype such user defined string, distance, etc.
Objects has the same relationship to a class that a variable has to a datatype. Object is an instance of a class. Class is a definition, objects are used in the program that enables you to store data and use them.
To understand the various syntactical requirements for implementing a class, let us take an example.
// distance.cpp
#include<iostream.h>
class distance
{ private:
int feet;
int inches;
public:
void setdistance(int a, int b)
{ feet=a;
inches=b;
}
void display()
{ cout<<feet<<”ft”<<inches<<”inches;}
};
void main()
{ distance d1;
d1. setdistance(10, 2);
d1.display();
}
In the above program, we have created a class called distance with two data members feet and inches and two functions or methods setdistance and display (). Classes are defined with the keyword class followed by the name of the class. The object of this class is d1. Every objects of a class have their own copy of data, but all the objects of a class share the functions. No separate copy of the methods is created for each and every object. Objects can access the data through the methods. To invloke a method, objectname.methodname is used. The methods defined in the class can only be invoked by the objects of the class. Without an object, methods of the function cannot be invoked.
You can see in the above program, the data elements feet and inches are defined as private. The methods are defined as public. These are known as access specifiers. Data and functions in a class can have any one access specifiers: private, public and protected. Protected access specifier we will discuss later in unit 7 along with inheritance. Private data and functions can be accessed only by the member functions of the class. None of the external functions can access the private data and member functions. Public data and member functions can be accessed through the objects of the class externally from any function. However to access these data and functions, you need to have an object of that class. This feature of object oriented programming is sometimes referred to as data hiding. The data is hidden from accidently getting modified by an unknown function. Only a fixed set of functions can access the data. In a class, the data and functions are private by default so private keyword can be omitted.
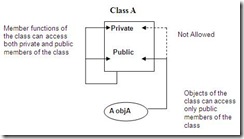
Fig. 5.1: Access specifiers in a class
Certain object oriented programming languages refer to methods as messages. Thus d1.display() is a message to d1 to display itself
Usually, data are declared as private and functions as public. However, in certain case you may even declare private functions and public data.
Please note that the class definition has a semicolon in the end.
Array of objects can also be created. It is similar to array of structures.
distance d[10]; creates a array of objects of type distance. If the first distance object accesses the member function display you can use d[0].display()
Let us see how we can perform operations on the objects data. We will see a program which implements an add member function to the distance class. We can do this in two ways. The prototypes of the function is shown below:
void add(distance, distance);
or
distance add(distance);
The first function takes two distance objects as arguments and stores the result in the object invoking the member function. It can be implemented as follows:
//adddist1.cpp
#include<iostream.h>
class distance
{ private:
int feet;
int inches;
public:
void setdistance(int a, int b)
{ feet=a;
inches=b;
}
void display()
{ cout<<feet<<”ft”<<inches<<”inches;}
void add(distance dist1, dist2)
{ feet=dist1.feet+dist2.feet;
inches=dist1.inches+dist2.inches;
if (inches>12)
{ feet++;
inches=inches-12;
}
}
};
void main()
{ distance d1,d2,d3;
d1. setdistance(10, 2);
d2.setdistance(2,4);
d3.add(d1,d2);
d3.display();
}
In the above program, object d3 invokes the add function so the feet and inches refers the invoking object’s data members. After adding the respective data of d1 and d2 it is stored in d3 object.
Alternatively, the above program can be implemented in a different way as shown below
//adddist2.cpp
#include<iostream.h>
class distance
{ private:
int feet;
int inches;
public:
void setdistance(int a, int b)
{ feet=a;
inches=b;
}
void display()
{ cout<<feet<<”ft”<<inches<<”inches;}
distance add(distance dist)
{ distance temp;
temp.feet=dist.feet+feet;
temp.inches=dist.inches+inches;
if (temp.inches>12)
{ temp.feet++;
temp.inches=temp.inches-12;
}
return temp;
}
};
void main()
{ distance d1,d2,d3;
d1. setdistance(10, 2);
d2.setdistance(2,4);
d3 =d1.add(d2);
d3.display();
}
In the above program, we have implemented the add function which is invoked by d1 object and d2 is passed as an argument. Since the calling objects data are passed automatically, d1 objects data members can be accessed in the function. They are referred directly as feet and inches without the objectname (as it is implicit in the member function). The add function stores the summed value in a temporary variable and then returns it to the calling program.
Self Assessment Questions
1. Private data members can be accessed only by the __________ of the class
2. Public data members can be accessed directly in the main function without an object. True/False
3. Every object of a class has its own copy of the data and functions. True/False
5.3 Constructors and Destructors
Constructors and destructors are very important components of a class. Constructors are member functions of a class which have same name as the class name. Constructors are called automatically whenever an object of the class is created. This feature makes it very useful to initialize the class data members whenever a new object is created. It also can perform any other function that needs to be performed for all the objects of the class without explicitly specifying it.
Destructors on the other hand are also member functions with the same name as class but are prefixed with tilde (~) sign to differentiate it from the constructor. They are invoked automatically whenever the object’s life expires or it is destroyed. It can be used to return the memory back to the operating system if the memory was dynamically allocated. We will see dynamic allocation of memory in Unit 7 while discussing pointers.
The following program implements the constructor and destructors for a class
// constdest.cpp
# include<iostream.h>
class sample
{ private:
int data;
public:
sample() {data=0; cout<<”Constructor invoked”<<endl;}
~sample() {cout<<”Destructor invoked”;}
void display()
{ cout<<”Data=”<<data<<endl;}
};
void main()
{ sample obj1;
obj.display();
}
If you run the above program you will get the output as follows:
Constructor invoked
Data=0
Destructor invoked
When object of sample class, obj is created, automatically the constructor is invoked and data is initialized to zero. When the program ends the object is destroyed which invokes the destructor. Please note that both the constructor and destructor is declared as public and they have no return value. However, constructors can have arguments and can be overloaded so that different constructors can be called depending upon the arguments that is passed. Destructors on the other hand cannot be overloaded and cannot have any arguments. The following program implements the overloaded constructors for the distance class.
//overloadconst.cpp
#include<iostream.h>
class distance
{ private:
int feet;
int inches;
public:
distance()
{ feet=0;
inches=0;}
distance(int f, int i)
{ feet=f;
inches=i;}
void display()
{ cout<<feet<<”ft”<<inches<<”inches;}
};
void main()
{ distance d1, d2(10,2);
d1.display();
d2.display()
}
In the above program, the setdistance function is replaced with the two constructors. These are automatically invoked. When object d1 is created the first constructor distance() is invoked. When object d2 is created the second constructor distance (int f, int i) is invoked as two arguments are passed. Please note that to invoke a constructor with arguments, argument values have to be passed along with the object name during declaration.
Self Assessment Questions
1. Constructors are member functions of the class which is invoked when ________ is created.
2. Destructors have same name as a class but are prefixed with ________ sign
3. Destructors can be overloaded. True/False
4. Constructors can be overloaded. True/False
5.4 Static variables and Functions in class
We have seen that when an object is created, a separate copy of data members is created for each and every object. But static data member in a class is an exception. Static data member of the class is one data member that is common for all the objects of the class and are accessible for the class. It is useful to store information like count of number of objects created for a particular class or any other common data about the class. Static data member is declared by prefixing the keyword static.
We have learnt that to invoke a member function of a class, you need to have object. Suppose we have a static data member which keeps track of the total objects created and there is a showtotal function which displays the total value. We will not be able to know the total if there are no objects which is a serious limitation. Static functions are the solution for this. Static functions are special type of functions which can be invoked even without an object of the class. Static functions are also defined by prefixing the keyword static. It is called using classname followed by scope resolution operator and function name. The following program implements the static data and functions
//classstatic.cpp
# include <iostream.h>
class A {
static int total;
int id;
public:
A()
{ total++;
id=total;
}
~A()
{ total--;
cout<<"n destroying ID number="<<id;
}
static void showtotal()
{cout<<"endl "<<total;}
void showid()
{cout<<"endl "<<id;}
};
int A::total=0;
void main()
{
A::showtotal();
A a1,a2;
A::showtotal();
a1.showid();
a2.showid();
}
The output of the above program
0
2
1
2
In the above program total is an static variable which is shared by all objects of the class A. The static variables of the class have to be explicitly initialised outside the class using the class name followed by scope resolution operator (::) and static variable name.
Please note that you have to specify the datatype but need not specify the keyword static during initialization. The variable total is incremented in the constructor which will increment the total whenever a new object is created. Similarly, it is decremented through the destructor when the object is destroyed. The function showtotal is a static function which is invoked without the object and using the classname.
The fig 5.2 summarises member functions and data of a class
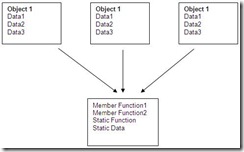
Fig. 5.2: Data and Functions in a class
Self Assessment Questions
1. Static data member of a class can be modified by all objects of the class. True/False
2. Static functions are accessed using __________
3. Static data need not be initialized. True/False
Summary
Class enable user to create user-defined datatypes and define their functionality. Objects are instances of classes. Class can contain both data and functions. Class data can be initialized using constructors which are invoked when the objects of a class are created. Destructors are member functions which are invoked when the objects are destroyed. Member functions can be accessed only through the objects of the class. Static functions can be accessed without objects. Static data can be accessed by all the objects of the class and only one copy of this data is maintained.
Terminal Questions
1. Implement a class stack which simulates the operations of the stack allowing LIFO operations. Also implement push and pop operations for the stack.
2. Write the output of the following program
# include<iostream.h>
class A
{ int a;
static int s;
public:
A()
{a=0; cout<<”Default Constructor Invoked”<<endl;}
A(int i)
{a=i; cout<<”Overloaded constructor Invoked”<<endl;s=s+a;}
void display()
{cout<<a<<endl<<s<<endl;}
}
int A::s=0;
void main()
{
A obj1(12);
obj1.display();
A obj2(10);
obj2.display();
}
3. Implement a class called employee that contains name, employee number and department code. Include a member function getdata() to get data from user and store it in the object. Write a program to test the class and it should store information of 100 employees .
Answers to SAQs in 5.2
1. member functions
2. False
3. False, only separate copy of data, but not functions
Answers to SAQs in 5.3
1. Object
2. Tilde or ~
3. False
4. True
Answers to SAQs in 5.4
1. True
2. class name and scope resolution operator
3. False
Answers to TQs
1. //stack.cpp
# include <iostream.h>
# define size 100
class stack
{ int stck[size];
int top;
public:
stack() {top=0;
cout <<"stack initialised"<<endl;}
~stack() {cout <<"stack destroyed"<<endl;}
void push(int i);
int pop();
};
void stack::push(int i)
{
if (top==size)
{ cout <<"stack is full";
return;
}
stck[top]=i;
top++;
}
int stack ::pop()
{ if (top==0) {
cout << "stack underflow" ;
return 0;
}
top--;
return stck[top];
}
void main()
{ stack a,b;
a.push(1);
b.push(2);
a.push(3);
b.push(4);
cout<<a.pop() << " ";
cout<<a.pop()<<" ";
cout<<b.pop()<< " ";
cout<<b.pop()<<endl;
}
2. Overloaded Constructor Invoked
12
12
Overloaded constructor Invoked
10
22
4. //arrobj.cpp
# include <iostream.h>
class employee
{ private:
int empid;
char name[35];
int deptcode;
public:
void getdata()
{ cout<<”enter employee code”;
cin>>empid;
cout<<”enter employee name”;
cin>>name;
cout<<”enter department code”;
cin>>deptcode;}
};
void main()
{ int i;
employee obj[100];
cout<<”enter employee data”;
for(i=0;i<100;i++)
{ obj[i].getdata();
}
}
Structure
5.1 Introduction
5.2 Creating classes and objects
Self Assessment Questions
5.3 Constructors and Destructors
Self Assessment Questions
5.4 Static variables and Functions in class
Self Assessment Questions
Summary
Terminal Questions
5.1 Introduction
This unit has the following objectives
· To understand what are classes and Objects and learn to implement them
· To learn to use constructors and destructors in a class
· To understand static variables and functions with respect to a class
5.2 Creating classes and objects
In the unit 1, we had a brief discussion of objects and classes. In this section, we will see how we can implement them. Classes provide users a method to create user defined datatypes. We have seen two types of user defined datatypes structures and enumeration in the last unit. Both are primarily used for modeling data only. In several real life situation, you would require to model functionality of the datatype along with the data. Classes enable you to bind data and functions that operate on data together. However, in C++ you can use structures same as classes by binding functions with data. But usually the structures are only used when you want to group data and classes when you want to group both data and functions.
Classes can model either physical or real things such as employee, product, customer etc or it can model a new datatype such user defined string, distance, etc.
Objects has the same relationship to a class that a variable has to a datatype. Object is an instance of a class. Class is a definition, objects are used in the program that enables you to store data and use them.
To understand the various syntactical requirements for implementing a class, let us take an example.
// distance.cpp
#include<iostream.h>
class distance
{ private:
int feet;
int inches;
public:
void setdistance(int a, int b)
{ feet=a;
inches=b;
}
void display()
{ cout<<feet<<”ft”<<inches<<”inches;}
};
void main()
{ distance d1;
d1. setdistance(10, 2);
d1.display();
}
In the above program, we have created a class called distance with two data members feet and inches and two functions or methods setdistance and display (). Classes are defined with the keyword class followed by the name of the class. The object of this class is d1. Every objects of a class have their own copy of data, but all the objects of a class share the functions. No separate copy of the methods is created for each and every object. Objects can access the data through the methods. To invloke a method, objectname.methodname is used. The methods defined in the class can only be invoked by the objects of the class. Without an object, methods of the function cannot be invoked.
You can see in the above program, the data elements feet and inches are defined as private. The methods are defined as public. These are known as access specifiers. Data and functions in a class can have any one access specifiers: private, public and protected. Protected access specifier we will discuss later in unit 7 along with inheritance. Private data and functions can be accessed only by the member functions of the class. None of the external functions can access the private data and member functions. Public data and member functions can be accessed through the objects of the class externally from any function. However to access these data and functions, you need to have an object of that class. This feature of object oriented programming is sometimes referred to as data hiding. The data is hidden from accidently getting modified by an unknown function. Only a fixed set of functions can access the data. In a class, the data and functions are private by default so private keyword can be omitted.
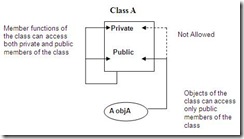
Fig. 5.1: Access specifiers in a class
Certain object oriented programming languages refer to methods as messages. Thus d1.display() is a message to d1 to display itself
Usually, data are declared as private and functions as public. However, in certain case you may even declare private functions and public data.
Please note that the class definition has a semicolon in the end.
Array of objects can also be created. It is similar to array of structures.
distance d[10]; creates a array of objects of type distance. If the first distance object accesses the member function display you can use d[0].display()
Let us see how we can perform operations on the objects data. We will see a program which implements an add member function to the distance class. We can do this in two ways. The prototypes of the function is shown below:
void add(distance, distance);
or
distance add(distance);
The first function takes two distance objects as arguments and stores the result in the object invoking the member function. It can be implemented as follows:
//adddist1.cpp
#include<iostream.h>
class distance
{ private:
int feet;
int inches;
public:
void setdistance(int a, int b)
{ feet=a;
inches=b;
}
void display()
{ cout<<feet<<”ft”<<inches<<”inches;}
void add(distance dist1, dist2)
{ feet=dist1.feet+dist2.feet;
inches=dist1.inches+dist2.inches;
if (inches>12)
{ feet++;
inches=inches-12;
}
}
};
void main()
{ distance d1,d2,d3;
d1. setdistance(10, 2);
d2.setdistance(2,4);
d3.add(d1,d2);
d3.display();
}
In the above program, object d3 invokes the add function so the feet and inches refers the invoking object’s data members. After adding the respective data of d1 and d2 it is stored in d3 object.
Alternatively, the above program can be implemented in a different way as shown below
//adddist2.cpp
#include<iostream.h>
class distance
{ private:
int feet;
int inches;
public:
void setdistance(int a, int b)
{ feet=a;
inches=b;
}
void display()
{ cout<<feet<<”ft”<<inches<<”inches;}
distance add(distance dist)
{ distance temp;
temp.feet=dist.feet+feet;
temp.inches=dist.inches+inches;
if (temp.inches>12)
{ temp.feet++;
temp.inches=temp.inches-12;
}
return temp;
}
};
void main()
{ distance d1,d2,d3;
d1. setdistance(10, 2);
d2.setdistance(2,4);
d3 =d1.add(d2);
d3.display();
}
In the above program, we have implemented the add function which is invoked by d1 object and d2 is passed as an argument. Since the calling objects data are passed automatically, d1 objects data members can be accessed in the function. They are referred directly as feet and inches without the objectname (as it is implicit in the member function). The add function stores the summed value in a temporary variable and then returns it to the calling program.
Self Assessment Questions
1. Private data members can be accessed only by the __________ of the class
2. Public data members can be accessed directly in the main function without an object. True/False
3. Every object of a class has its own copy of the data and functions. True/False
5.3 Constructors and Destructors
Constructors and destructors are very important components of a class. Constructors are member functions of a class which have same name as the class name. Constructors are called automatically whenever an object of the class is created. This feature makes it very useful to initialize the class data members whenever a new object is created. It also can perform any other function that needs to be performed for all the objects of the class without explicitly specifying it.
Destructors on the other hand are also member functions with the same name as class but are prefixed with tilde (~) sign to differentiate it from the constructor. They are invoked automatically whenever the object’s life expires or it is destroyed. It can be used to return the memory back to the operating system if the memory was dynamically allocated. We will see dynamic allocation of memory in Unit 7 while discussing pointers.
The following program implements the constructor and destructors for a class
// constdest.cpp
# include<iostream.h>
class sample
{ private:
int data;
public:
sample() {data=0; cout<<”Constructor invoked”<<endl;}
~sample() {cout<<”Destructor invoked”;}
void display()
{ cout<<”Data=”<<data<<endl;}
};
void main()
{ sample obj1;
obj.display();
}
If you run the above program you will get the output as follows:
Constructor invoked
Data=0
Destructor invoked
When object of sample class, obj is created, automatically the constructor is invoked and data is initialized to zero. When the program ends the object is destroyed which invokes the destructor. Please note that both the constructor and destructor is declared as public and they have no return value. However, constructors can have arguments and can be overloaded so that different constructors can be called depending upon the arguments that is passed. Destructors on the other hand cannot be overloaded and cannot have any arguments. The following program implements the overloaded constructors for the distance class.
//overloadconst.cpp
#include<iostream.h>
class distance
{ private:
int feet;
int inches;
public:
distance()
{ feet=0;
inches=0;}
distance(int f, int i)
{ feet=f;
inches=i;}
void display()
{ cout<<feet<<”ft”<<inches<<”inches;}
};
void main()
{ distance d1, d2(10,2);
d1.display();
d2.display()
}
In the above program, the setdistance function is replaced with the two constructors. These are automatically invoked. When object d1 is created the first constructor distance() is invoked. When object d2 is created the second constructor distance (int f, int i) is invoked as two arguments are passed. Please note that to invoke a constructor with arguments, argument values have to be passed along with the object name during declaration.
Self Assessment Questions
1. Constructors are member functions of the class which is invoked when ________ is created.
2. Destructors have same name as a class but are prefixed with ________ sign
3. Destructors can be overloaded. True/False
4. Constructors can be overloaded. True/False
5.4 Static variables and Functions in class
We have seen that when an object is created, a separate copy of data members is created for each and every object. But static data member in a class is an exception. Static data member of the class is one data member that is common for all the objects of the class and are accessible for the class. It is useful to store information like count of number of objects created for a particular class or any other common data about the class. Static data member is declared by prefixing the keyword static.
We have learnt that to invoke a member function of a class, you need to have object. Suppose we have a static data member which keeps track of the total objects created and there is a showtotal function which displays the total value. We will not be able to know the total if there are no objects which is a serious limitation. Static functions are the solution for this. Static functions are special type of functions which can be invoked even without an object of the class. Static functions are also defined by prefixing the keyword static. It is called using classname followed by scope resolution operator and function name. The following program implements the static data and functions
//classstatic.cpp
# include <iostream.h>
class A {
static int total;
int id;
public:
A()
{ total++;
id=total;
}
~A()
{ total--;
cout<<"n destroying ID number="<<id;
}
static void showtotal()
{cout<<"endl "<<total;}
void showid()
{cout<<"endl "<<id;}
};
int A::total=0;
void main()
{
A::showtotal();
A a1,a2;
A::showtotal();
a1.showid();
a2.showid();
}
The output of the above program
0
2
1
2
In the above program total is an static variable which is shared by all objects of the class A. The static variables of the class have to be explicitly initialised outside the class using the class name followed by scope resolution operator (::) and static variable name.
Please note that you have to specify the datatype but need not specify the keyword static during initialization. The variable total is incremented in the constructor which will increment the total whenever a new object is created. Similarly, it is decremented through the destructor when the object is destroyed. The function showtotal is a static function which is invoked without the object and using the classname.
The fig 5.2 summarises member functions and data of a class
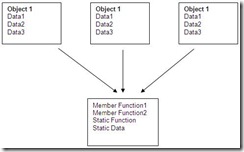
Fig. 5.2: Data and Functions in a class
Self Assessment Questions
1. Static data member of a class can be modified by all objects of the class. True/False
2. Static functions are accessed using __________
3. Static data need not be initialized. True/False
Summary
Class enable user to create user-defined datatypes and define their functionality. Objects are instances of classes. Class can contain both data and functions. Class data can be initialized using constructors which are invoked when the objects of a class are created. Destructors are member functions which are invoked when the objects are destroyed. Member functions can be accessed only through the objects of the class. Static functions can be accessed without objects. Static data can be accessed by all the objects of the class and only one copy of this data is maintained.
Terminal Questions
1. Implement a class stack which simulates the operations of the stack allowing LIFO operations. Also implement push and pop operations for the stack.
2. Write the output of the following program
# include<iostream.h>
class A
{ int a;
static int s;
public:
A()
{a=0; cout<<”Default Constructor Invoked”<<endl;}
A(int i)
{a=i; cout<<”Overloaded constructor Invoked”<<endl;s=s+a;}
void display()
{cout<<a<<endl<<s<<endl;}
}
int A::s=0;
void main()
{
A obj1(12);
obj1.display();
A obj2(10);
obj2.display();
}
3. Implement a class called employee that contains name, employee number and department code. Include a member function getdata() to get data from user and store it in the object. Write a program to test the class and it should store information of 100 employees .
Answers to SAQs in 5.2
1. member functions
2. False
3. False, only separate copy of data, but not functions
Answers to SAQs in 5.3
1. Object
2. Tilde or ~
3. False
4. True
Answers to SAQs in 5.4
1. True
2. class name and scope resolution operator
3. False
Answers to TQs
1. //stack.cpp
# include <iostream.h>
# define size 100
class stack
{ int stck[size];
int top;
public:
stack() {top=0;
cout <<"stack initialised"<<endl;}
~stack() {cout <<"stack destroyed"<<endl;}
void push(int i);
int pop();
};
void stack::push(int i)
{
if (top==size)
{ cout <<"stack is full";
return;
}
stck[top]=i;
top++;
}
int stack ::pop()
{ if (top==0) {
cout << "stack underflow" ;
return 0;
}
top--;
return stck[top];
}
void main()
{ stack a,b;
a.push(1);
b.push(2);
a.push(3);
b.push(4);
cout<<a.pop() << " ";
cout<<a.pop()<<" ";
cout<<b.pop()<< " ";
cout<<b.pop()<<endl;
}
2. Overloaded Constructor Invoked
12
12
Overloaded constructor Invoked
10
22
4. //arrobj.cpp
# include <iostream.h>
class employee
{ private:
int empid;
char name[35];
int deptcode;
public:
void getdata()
{ cout<<”enter employee code”;
cin>>empid;
cout<<”enter employee name”;
cin>>name;
cout<<”enter department code”;
cin>>deptcode;}
};
void main()
{ int i;
employee obj[100];
cout<<”enter employee data”;
for(i=0;i<100;i++)
{ obj[i].getdata();
}
}
No comments:
Post a Comment